Shell Scripting
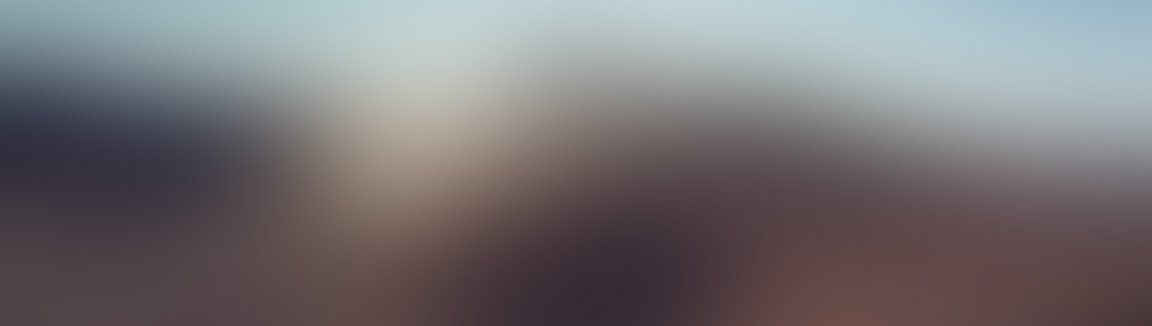
What is shell scripting?
Shell scripting a way to automate tasks in Unix-like operating systems by writing scripts (sometimes referred to as small programs).
What is a shell script?
A shell script is a text file with a list of UNIX commands written in a shell language, such as Bash. Instead of typing commands manually one by one in the terminal, you can save them in a script and run that script to execute all the commands automatically. Shell scripts use plain text with commands written similarly to how you would type them in the terminal. Scripts can use variables to store data and can use structures like loops and conditionals to make decisions and repeat actions.
Why should I use shell scripting?
Shell scripting enhances productivity by allowing users to bundle a series of commands into a single, executable file, which can be run with a single command. Using shell scripts can be more efficient, saving you time by running multiple commands with a single script. Shell scripts are also good for consisitency and reproducibility. By using a shell script, you ensure that the commands are run the same way every time. In addition, shell scripts are helpful because they can be re-used to perform routine tasks across different projects or systems.
Creating and Running a Script
- To create a shell script, create a text file with the desired name but use a
.sh
extention instead of.txt
. The.sh
is the standard file extension for shell scripts. This extension indicates that the file is a bash script. For example, to create a script named "practice.sh", we would run: - After opening the .sh file in a text editor (like nano), we need to add
#!/bin/bash
to the first line. The#!
is known as a shebang or a hashbang. The#!
tells our computer what system to run the script with (in this case, Bash). The first line in your script should be: - Now, we can add our first command anywhere below the first line. For example, add
echo "This is a bash script!"
. When the script runs,echo
will printThis is a bash script!
. For example, your script should now look like this: - Within a script, we can write "comments". Comments are notes in a script that do not get run. A comment is indicated by the
#
character. For example, we can write a comment adding some information about the purpose of our script: - Now save the script with
Ctrl + O
and exit withCtrl + X
. - In order to run the script, we need to specify where our script lives. To do this, we can either specify the absolute path, for example:
Users/username/[path]/practice.sh
, or the relative path,./practice.sh
(remember that.
indicates the current working directory) in the command line: - However, you will not be able to run the command above until we give it the correct permissions. To see the file's permissions, run
ls -l
, which prints detailed information about the contents of the current working directory. - The first column of the output shows file permissions. The permissions for practice.sh may look like
-rw-r--r--
. To change the permissions so the script can be run by all users, run the following code in the command line: - Re-run
ls -l
. The permissions for practice.sh should now look like-rwxr-xr-x
. Now that we have executable permissions for that file, we can run it:
$ nano practice.sh
#!/bin/bash
#!/bin/bash
echo "This is a bash script!"
#!/bin/bash
# This script was made for practice
echo "This is a bash script!"
$ ./practice.sh
bash: demo.sh: command not found...
ls -l
$ chmod +x practice.sh
$ ./practice.sh
- For more information about permissions, please visit the Permissions page.
Shell Variables
See the Bash/Command Line page for more information about variables.
Just like in the command line, we can save information under variables within scripts so that we can access the information later. To set a variable, pick a name (containing only letters, numbers, and underscores). For this example, let's use VAR
. Note: shell variable names are traditionally capitalized, but do not have to be. Remember, to access a value stored in a variable, we precede the variable with a $
. Let's edit our script to add a variable:
$ nano practice.sh
#!/bin/bash
# This script was made for practice
echo "This is a bash script!"
# Declare our new variable
VAR = "I am great at this!"
# Print the variable
echo $VAR2
In addition to declaring variables within a script, we can also pass arguments into the script from the command line. For example:
$ nano practice.sh "I'm a pro."
#!/bin/bash
# This script was made for practice
echo "This is a bash script!"
# Declare our new variable
VAR = "I am great at this!"
# Print the variable
echo $VAR
# Print variable from command line
echo $1
In this example, we passed the argument "I'm a pro." into our script. Because "I'm a pro." is our first argument, we will access the variable with $1
. If we had two arguments, the second argument would be accessed with $2
, and so on. This script should output:
This is a bash script!
I am great at this!
I'm a pro.
- $1, $2, etc., refer to specific arguments (e.g., $1 for the first argument).
$@
refers to all command-line arguments.- Place variables in quotes if their values might contain spaces to ensure they are correctly interpreted.
Here is an example of a for loop in a script:
$ nano loop.sh
#!/bin/bash
# This is a for loop
for VAR in a b c
do
echo $VAR
done
The loop runs over once for each item after the word in
. Each iteration (i.e., run or loop), the variable $VAR is set to a particular value. The value of $VAR
is a
in the first iteratation, b
in the second iteratation, and c
in the third iteratation.
For more infromation, see Andy's Brain Book Scripting Tutorial for more information and examples.